An overview of the Fibonacci series in C++
- Akshay Sharma
- Jan 17, 2023
- 4 min read
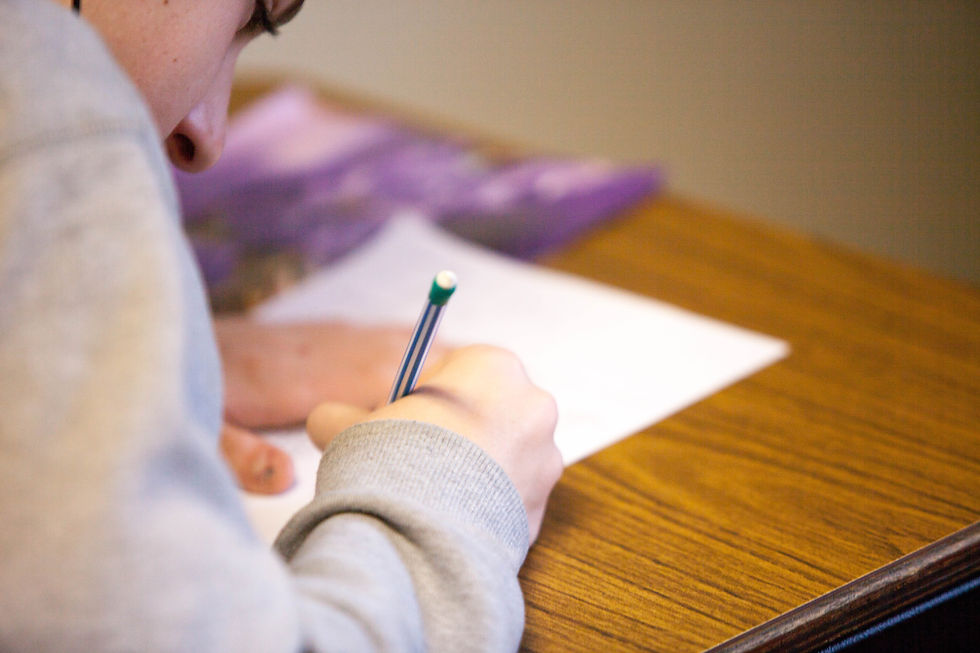
As a budding programmer, your main focus should always be to have a stronghold on programming fundamentals.
You should be well-versed in all the programming concepts rather than just learning new languages every time.
One such fundamental concept of programming is the Fibonacci series. The main reason behind the significant popularity of the Fibonacci series is that though it seems a basic question, a lot of thought and logic is put into the concept.
Moreover, you can use multiple methods to print a Fibonacci series in C++ or any other language.
With that being said, Are you aware of all the methods that you can use to print a Fibonacci series? Do you know which method is optimal to print a Fibonacci series?
Well, if you are not aware, keep reading to know all the possible methods that you can use to print a Fibonacci series in C++.
Wait! Basics first! Let's understand the meaning of the Fibonacci series in detail.
What Is A Fibonacci Series?
A Fibonacci series is defined as a number series derived by adding the two previous elements of the series from the current number. Here, you need to know that the beginning elements of the Fibonacci series are always 1 and 0.
To find the Fibonacci series, generally, the following formula is used:
f(n) = f(n-1) + f(n-2)
Always remember that the values of F(0) and F(1) will always be 0 and 1 respectively.
If you are still confused about the concept of the Fibonacci series, consider the following example:
Input: n= 6
The output series will be 0 1 1 2 3 5
Explanation:
0+1= 1
1+1= 2
1+2= 3
3+2= 5
This is how a Fibonacci series works. Let's now explore all the possible methods to print the Fibonacci series in C++.
Approaches to Find Fibonacci Series In C++
The following approaches can be used to print the Fibonacci series in C++:
Iterative method
Using dynamic programming
Using recursion
With the help of a matrix.
Let's go through each of them in detail.
Iterative Method
In this method, you will have to use the while loop to sum the number. The Iterative method is one of the most used and basic methods to calculate the Fibonacci series in C++. For this, you will first have to initialise the starting two numbers i.e. 0 and 1. After initialising, you will have to use a while loop and sum up the two previous numbers from the current index.
Simultaneously, you need to swap between the first and second and second and third numbers
Complexity Analysis
Time Complexity: In an iterative approach, the time complexity is O(n).
Space complexity: In an iterative approach, the space complexity is O(1).
Dynamic Programming
In the iterative or recursive approach, the time complexity of the program may increase. Therefore, to optimise the complexity, dynamic programming is used.
The following steps are used in dynamic programming.
Declare and initialise an array with size N.
Now, declare array[0]= 0 and array[1]= 1.
After this, you will have to iterate the whole array from index 2 and update the value of the array as Array[i]= array[i-2] + array[i-1]
Finally, return the final values of array[n].
Complexity Analysis
Time Complexity: For dynamic programming, the time complexity is calculated as O(n).
Space complexity: For dynamic programming, space complexity is O(n).
Using Recursion
Next method that you can use to find Fibonacci series in C++ is the recursion method. In this method, the main logic is to use the fact that a Fibonacci series is obtained by summing up previous elements of the series.
Following steps are included in the process:
Find the number for which you need to find the Fibonacci series.
Iterate the values from N to 1 recursively. While iterating, following cases may occur:
Base case: Here, the value of recursion is less than 1. The function here will return 1.
Recursive Case: Here, the base case condition is not Satisfied. Therefore, you will have to recursively iterate the array.
Return statement: after every iteration, you are required to return the previous values.
Complexity Analysis
Time Complexity: In the recursion method, the time complexity is O(2^n).
Space complexity: In the recursion method, the space complexity is O(1).
With The Help Of A Matrix
Another method that we can use to print the Fibonacci series in C++ is using a matrix. The logic behind this method is that if we attempt to multiply a matrix M= {{1, 1}, {1, 0}} to itself for n times, the resultant matrix will have (n+1) Fibonacci values as its element of a row or column.
Complexity Analysis
Time complexity: Here, the time complexity is calculated as O(n).
Space complexity: Now that we are not using any additional space, the space complexity will be equal to O(1).
You must know that although programming languages change, the basic concepts associated with coding remain the same.
So, now that we are discussing the Fibonacci series and since java is another most used language, let's understand how one can write a program to print the Fibonacci series in Java.
To print the Fibonacci series in Java, three methods can be used:
Iteration method
Recursion method
Dynamic programming
However, depending on the input method and type, you can choose the best and optimal method.
Conclusion
A Fibonacci series is a fundamental concept of programming and is often asked by interviewers to test your hold on the basics of programming.
When it comes to writing code to print the Fibonacci series in C++, multiple methods can be used including the iterative method, recursion method and dynamic programming methods.
However, each of these methods has its own time and space constraints which completely depend on the input value.
Therefore, while choosing a method to print the Fibonacci series in Java, C++ or any other programming language, make sure to take a look at the time and space constraints of the chosen method.
Comments